Arduino enthusiasts often seek effective ways to display data, and integrating an LCD screen is a popular method. In this guide, we will delve into the use of a 1602A LCD display with an Arduino, focusing on the I2C interface for streamlined communication. This optimized guide covers setup, coding, and troubleshooting to ensure your project succeeds.
Introduction to the 1602A LCD Display
The 1602A LCD display is a versatile, user-friendly screen featuring 16 columns and 2 rows of alphanumeric characters. This display is perfect for projects requiring simple text output. Its I2C interface simplifies wiring and reduces pin usage on your Arduino, making it an efficient choice for many applications.
Why Use I2C with the 1602A LCD Display?
Benefits of I2C:
- Fewer Pins Required: I2C uses only two pins (SDA and SCL) compared to the multiple pins needed for parallel communication.
- Simplified Wiring: Reduces clutter and potential errors in connections.
- Expandability: Allows multiple I2C devices on the same bus without additional pins.
Components Needed
- Arduino (e.g., Arduino Uno, Nano)
- 1602A LCD Display with I2C Interface
- Jumper wires
- Breadboard (optional)
Setting Up the 1602A LCD Display with Arduino
Step 1: Connecting the Hardware
- Connect the I2C module to the LCD:
- Attach the I2C module to the back of the 1602A LCD display if not already attached.
- Wire the LCD to the Arduino:
- GND: Connect to Arduino GND
- VCC: Connect to Arduino 5V
- SDA: Connect to Arduino A4 (for Arduino Uno/Nano)
- SCL: Connect to Arduino A5 (for Arduino Uno/Nano)
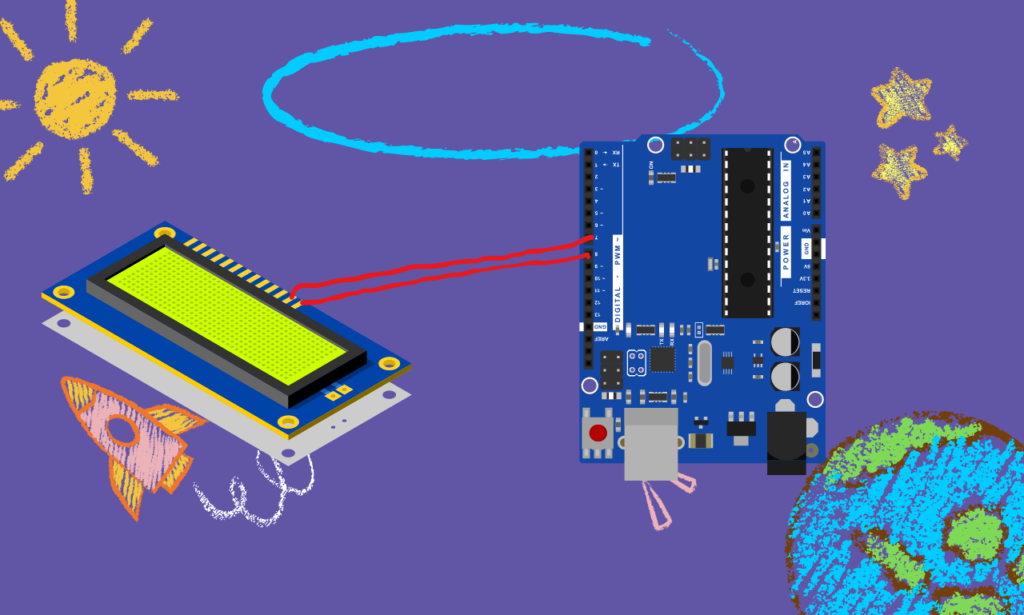
Step 2: Installing the Required Libraries
To use the 1602A LCD display with I2C, install the LiquidCrystal_I2C
library:
- Open the Arduino IDE.
- Go to Sketch > Include Library > Manage Libraries.
- In the Library Manager, search for
LiquidCrystal_I2C
and install it.
Step 3: Writing the Code
Here’s a basic code example to get you started with displaying text on your 1602A LCD using I2C:
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
// Initialize the library with the I2C address and LCD size
LiquidCrystal_I2C lcd(0x27, 16, 2); // 0x27 is a common I2C address for the module
void setup() {
lcd.begin(); // Initialize the LCD
lcd.backlight(); // Turn on the backlight
// Print a message to the LCD
lcd.setCursor(0, 0); // Set the cursor to column 0, row 0
lcd.print("Hello, World!");
lcd.setCursor(0, 1); // Set the cursor to column 0, row 1
lcd.print("Arduino LCD");
}
void loop() {
// Your code here (if any)
}
Step 4: Uploading and Testing
- Connect your Arduino to your computer using a USB cable.
- Select the correct board and port from the Tools menu in the Arduino IDE.
- Click the upload button to transfer the code to your Arduino.
- Once uploaded, you should see “Hello, World!” and “Arduino LCD” displayed on your 1602A LCD.
Troubleshooting Tips
- No Display: Ensure all connections are secure and correct. Check if the I2C address matches your module’s address (common addresses are 0x27 and 0x3F).
- Garbled Text: Adjust the contrast using the potentiometer on the I2C module.
- Library Issues: Ensure you have the correct and updated version of the
LiquidCrystal_I2C
library.
Conclusion
Integrating a 1602A LCD display with your Arduino via I2C simplifies your project’s wiring and conserves precious GPIO pins. By following this guide, you should have a functioning setup displaying custom messages on your LCD screen. Experiment with different messages and incorporate this display into your broader projects for enhanced data visualization.
Comments